I see that it is possible to sort a user query by the number of posts each user has, but is it possible to exclude users with zero posts from the result? In the Wp_User_Query class there is a pre_user_query
action, but query strings are a huge weak point, so I’m not sure what sort of filter action I’d want to use here.
WP_User_Query to exclude users with no posts
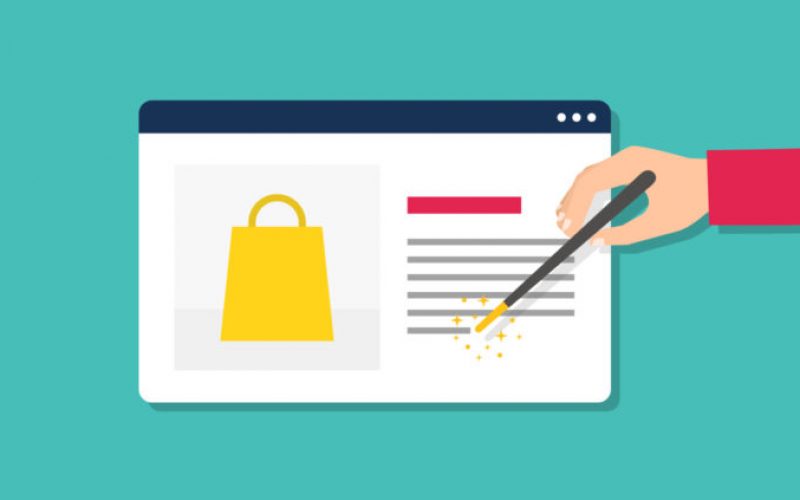
Well I have come up with 2 solutions.
Solution 1 – foreach loop and verify each user
This one is based off of @GhostToast’s solution, but with updated WordPress functions
Solution 2 – fancy pants
pre_user_query
actionThis is what I was thinking of when I posted my question once I found the
pre_user_query
action in theWP_User_Query
class. If you pass inpost_count
as yourorderby
parameter then some fancy SQL querying that I never would’ve figured out on my own happens to join the proper tables together. So what I did was copy that join statement and add it on to my own. This would be better if I could check for its presence first before adding it… perhaps I will use a string match in the future. But for now since I am the one setting up the query I know it isn’t there and I just won’t worry about it yet. So the code turned out like so:and then to use it
The idea for a
query_id
parameter is from An Introduction to WP_User_ClassWhich is also just a very good reference on
WP_User_Query
Since 4.4, you can simply use the `has_published_posts’ parameter.
Example:
has_published_posts
can be either true/false (or null), or an array of post types (like in this example).Note: I’m using transients here because this specific query can get pretty heavy depending on the system so it makes sense to store it for future uses.
Submitting as answer for closure: