I’m a newbie in WordPress. I have created a custom post type for videos but I do not know how to display the post type in a page. For example I want when a user adds a video, he won’t have to select a video template when publishing the video and when they open the published video, the page opens up with a video player instead of opening a page. I want something like a custom page with the video player ready that all I have to do is give the video player the url for the video.Already have the code for the video player. How can I do this?
WordPress Display Custom post types
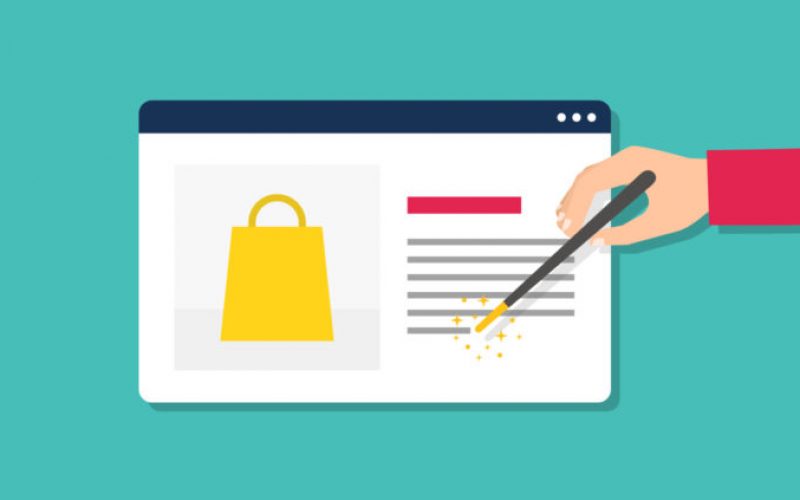
To make a default template file for all your custom post type posts or pages, you can name your template file
single-{your-cpt-name-here}.php
orarchive-{your-cpt-name-here}.php
and it will always default to this when viewing these posts or pages.So for example in
single-video.php
you could put:or instead make a custom query to shape your desired output:
In your custom loop like the example above, there’s lots of available template tags (like
the_content
) to choose from in WordPress.Write Code In Functions.php
Now Write This Code Where You Want To show
?>
After you created the CPT, do this for showing single posts of your CPT:
single.php
file in your template and rename it likesingle-{post_type}.php
(eg.single-movie.php
)You can get more details from this post
Now if you want to show a list of CPT you can use get_posts() with args:
$args = array(
...
'post_type' => 'movie'
)
Check this post for further details.