Is there any way to validate custom meta box fields without using javascript. If it doesn’t validate I want to stop the post from being saved to the database.
WordPress Custom Meta Boxes Validation
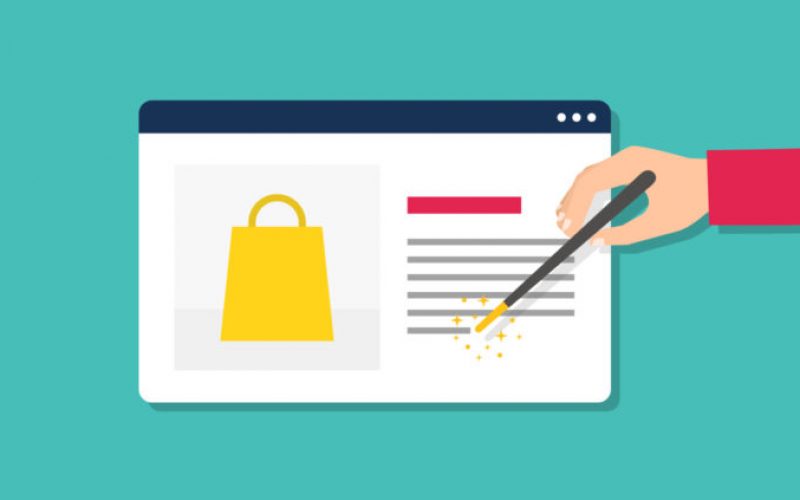
Is there any way to validate custom meta box fields without using javascript. If it doesn’t validate I want to stop the post from being saved to the database.
You must be logged in to post a comment.
Since the ‘save_post’ action is run AFTER publishing and updating, there’s really no way of validating custom keys without a hackish alternative.
However, I’m thinking you can mimic the functionality you want by employing ‘save_post’ in the way Viral suggested, but rather than interrupt or cancel the saving process upon a validation error, you can just delete the post altogether:
The only thing to watch out for with this approach is that it will run upon both Publishing and Updating as well. You may want to consider adding a check to ensure that the post is deleted only for newly published posts, and updated posts are rolled back to a previous version and the invalid revision is deleted.
The
wp_insert_post_data
filter is what you are looking for. Something like this should do the trick:Straight from the WP Codex @ http://codex.wordpress.org/Function_Reference/add_meta_box, you call the
save_post
hook and specify the function that will be run to validate/save your data:Then you define that function, which will automatically be passed the post id. Additionally, you can access the $_POST array to get the values in your metaboxes:
All of your routines to valid data will be done within this function. In the end, you will likely save the data using something like:
update_post_meta('meta_key', 'meta_value');