I want to prevent users from adding products from different categories into cart at once.
Say if the user navigates to another categories and tries to add product to cart, they get the cart cleared out first.
Please anybody know how i might approach this? I am a newbie at things like this.
Thanks
Woocommerce – Prevent Adding items from two different categories to cart
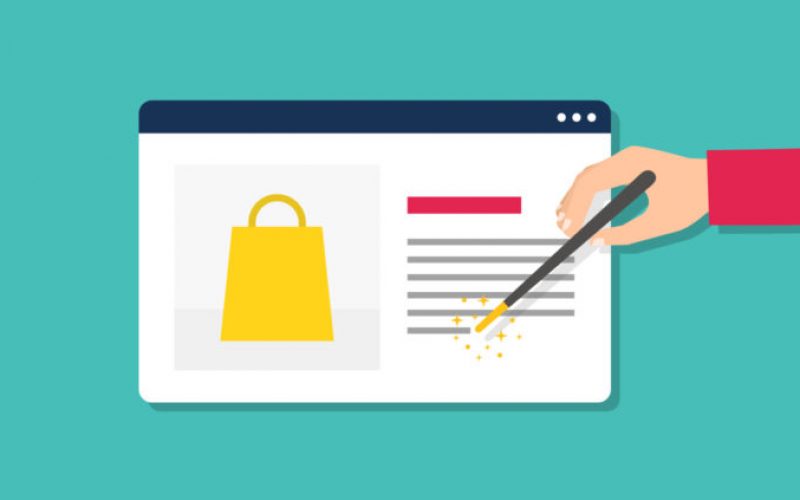
I’ve solved this before. My write-up is here.
It differs slightly from your request in that instead of clearing the cart when they try to add a second category it keeps the original item in the cart and displays a warning.
For posterity:
Here is my solution for this (You can replace ‘city’ by ‘product_cat’ to make it work for product categories: