I really like the WP_Image_Editor
class. I wanted to use it for some custom functionality I’m making, but I was wondering – Watermarking images – is there a way that I could use the objects created by WP_Image_Editor
to merge an image with a watermark? I cannot figure out any obvious way.
Watermarking Images with WordPress with WP_Image_Editor
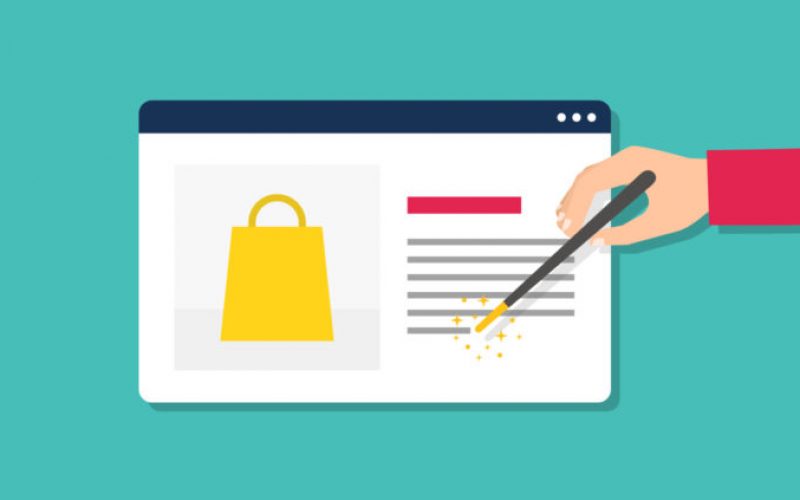
If you really want to use these classes the only way would be to extend both existing implementations
Wp_Image_Editor_Imagick
andWp_Image_Editor_GD
.Here’s an approach for the
Wp_Image_Editor_GD
:The implementation follows this example on php.net.
Now that you have your implementation you must add the new class to the stack of possible image editors using the filter
wp_image_editors
:Now it’s likely possible to get an instance of your custom editor when calling
wp_get_image_editor()
:You could also simply create the instance explicitly. Every other client, using
wp_get_image_editor()
won’t be aware of the methodstamp_watermark()
anyway.Some important notes:
Wp_Image_Editor_Gd
class.test()
andsupportet_mime_types()
according to this.I have had a lot of problems implementing David code. I had errors like “WP_IMAGE_EDITOR_GD” not found, “WatermarkImageEditor” not found…
And when I got it to work, it didn’t work with alpha channel png, so I lost a lot of hours making it work with them. So I will explain how I have it.
Put all this code in a php file, mine is called watermark.php.
Now, we need to register the filter. I am using it in my own plugin, so I have this code on my main plugin file, but you can also put it on other place like functions.php. Note that I am using require_once to load watermark.php, so watermark.php has to be in the same folder.
And the last step, call to stamp_watermark(). On this example, I am loading an image from disk, resizing it, putting the watermark, and saving it. Note that stamp_watermark() receives on the first parameter, the path or url of the watermark, the other 2 parameters are the optional margin.