I want to hide the WordPress post from archives page using the ‘private’ option. I only want the post to be hidden from archives but visible to public. How to I do it?
How to hide a post from archives
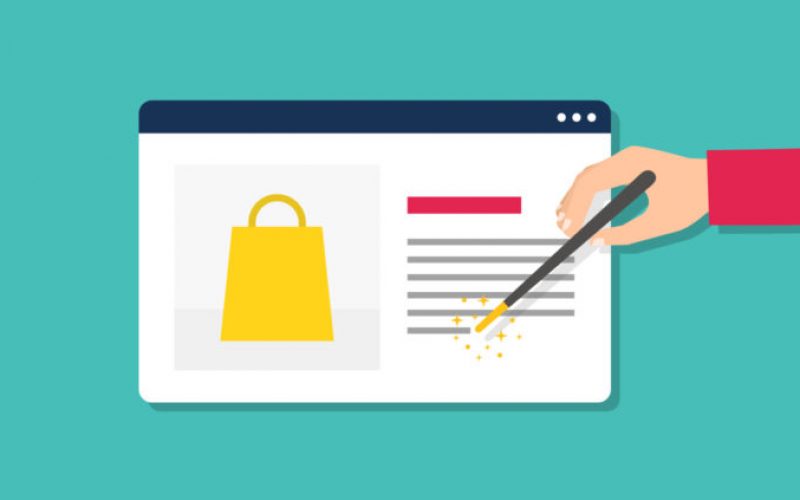
I want to hide the WordPress post from archives page using the ‘private’ option. I only want the post to be hidden from archives but visible to public. How to I do it?
Comments are closed.
You’ll want to filter the main query via
pre_get_posts
callback.If you’ll only want to handle one specific post in this manner, you can reference/exclude its post ID directly. If, however, you want this same behavior for any future private posts, then I would query all posts with
$post->post_status
ofprivate
, and exclude them.One post (where ID = 123):
Or, for all
private
posts:I think the easiest way would be to control the loop in the
archive.php
file, IF it’s just the one post you want to hide from the archives.Where 7 is the post_ID of the post you want to hide and the
-
sign designates you want to exclude it from the loop.Take a look at this link that explains the usage and arguments that can be passed, in case you want to go further then what your question suggests.
Here and here you can find alternate methods to accomplish what I mentioned.
— Update —
Given toscho’s comments I’ve dug around a bit and here is how you can use a filter on the
pre_get_posts()
: