I’m building a plugin and I want to add bits of javascript in the admin head but only for certain admin pages. I don’t mean pages as in a WordPress page that you create yourself but rather existing admin section pages like ‘Your Profile’, ‘Users’, etc. Is there a wp function specifically for this task? I’ve been looking and I can only find the boolean function is_admin
and action hooks but not a boolean function that just checks.
How can you check if you are in a particular page in the WP Admin section? For example how can I check if I am in the Users > Your Profile page?
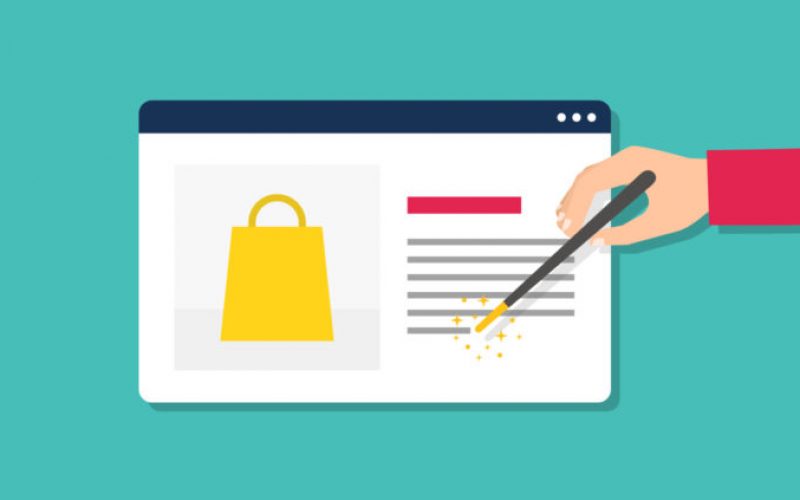
The way to do this is to use the ‘admin_enqueue_scripts’ hook to en-queue the files you need. This hook will get passed a $hook_suffix that relates to the current page that is loaded:
There is a global variable in wp-admin called $pagenow which holds name of the current page, ie edit.php, post.php, etc.
You can also check the $_GET request to narrow your location down further, for example:
The most comprehensive method is
get_current_screen
added in WordPress 3.1returns
To offer an alternative method/approach to the above question.
This method targets the specific pages more directly and avoids needing conditional logic inside your callback(because you’ve already made that distinction in the selected hook).
I find it weird that no one has mentioned the fact that the add_menu_page function returns an action hook which you can use to do certain actions only on those pages
If you need the $hook and you didn’t add the menu page yourself the doc is here
For instance the hook for a top level menu page is
The hook for a submenu page is
Following that logic, the hook for the user’s profile page is