I have that site : http://ougk.gr and I want on the navigation to have a link which points to the latest post of a specific category (full post with comments etc). How can I achieve that?
Get latest post link on WordPress
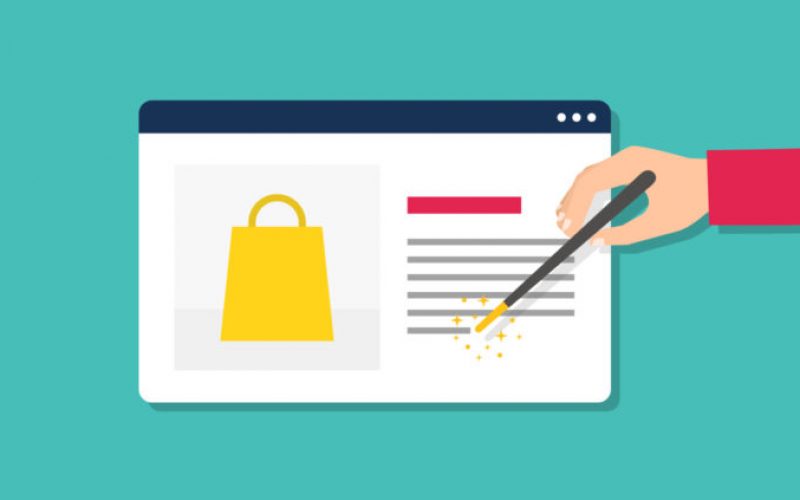
I have that site : http://ougk.gr and I want on the navigation to have a link which points to the latest post of a specific category (full post with comments etc). How can I achieve that?
You must be logged in to post a comment.
There are a few ways to do this. This one uses wp_get_recent_posts(), and prints a basic link:
Where
CAT_ID
is the id of the targeted category. For your situation the simple answer is to insert the link code just after the opening nav tag, as above.To place the link somewhere else in the nav, you’ll have to dive into some of the other functions called in the code you pasted. It might be a good idea to get your hands dirty..
You need ot get the title and perma link