I am performing an jquery ajax request inside my wordpress. This calls an internal php script.
This php script needs to be able to access certain wordpress features like… functions.php
which is simple for me to include.
What i cant do is access info like the current wordpress user, the $wpdb object.
My question is… is there some wordpress file which i can include which gives me access to all that data (and functions.php).
I hope you understand what i am accessing as i am aware that was probably THE crappest explaination in the world
😀
Creating an ajax call in wordpress. What do i have to include to access wordpress functions
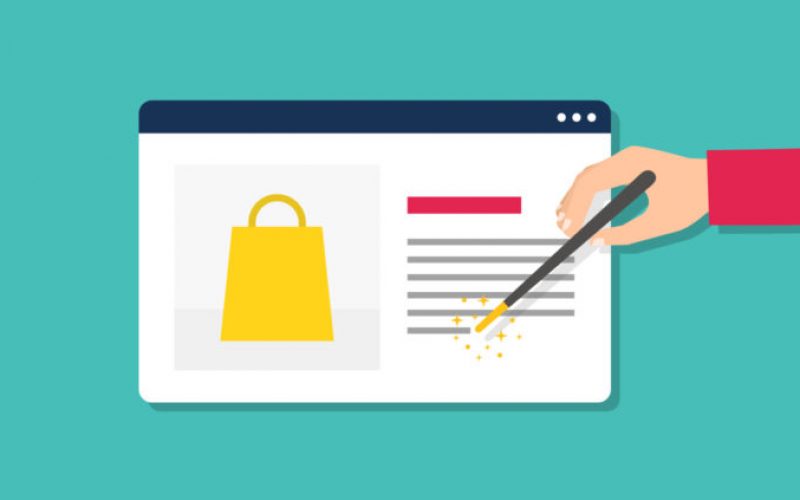
THE BAD WAY (as pointed out by others)
When I created some custom PHP to use with wordpress I included the
wp-load.php
file. Which then loads everything required, including$wpdb
.I found it was a decent starting point for a quick fix. However you have to remember this will load in a lot more functionality than you may actually require. Thus resulting in slower performance times.
THE GOOD WAY
Once functionality became more complex the ‘bad’ implementation wasn’t proving to be all that great. So I moved onto writing plugins instead. The WordPress codex contains good information on working with AJAX and plugins: http://codex.wordpress.org/AJAX_in_Plugins
In the most basic form you will need to register your AJAX hook:
You will also need the corresponding function (in this case
foo
):Then in your JavaScript you identify which hook to use with the
action
attribute but leave out thewp_ajax
part:I typically set up an action hook in
functions.php
to listen for AJAX requests based on a prefix, like ‘wp_ajax_*’. You will need to have a reference towp-load.php
in your javascript as well, which can be added usingwp_head
. Once this is set up, just use a variable called “action” in your AJAX request to indicate which function you want to use.Your Javascript request would look similar to the following:
AJAX requests in WordPress are typically sent via special hooks in the
admin-ajax.php
file on thewp-admin
directory. From there you’ll have access to all of WP functions.here is a good place to start
You shall never include neither wp-load.php, nor wp-config.php – this is a bad practice, and this article explains why: http://ottodestruct.com/blog/2010/dont-include-wp-load-please/. Also, WordPress.org team does not recommend including any WordPress core files directly.
Instead, you can utilise the functionality of admin-ajax.php – don’t be deceived by ‘admin’ part – it is used in both front-end and back-end scripts. Here is an example: http://wp.smashingmagazine.com/2011/10/18/how-to-use-ajax-in-wordpress/