Creating a post from data returned from HTML form
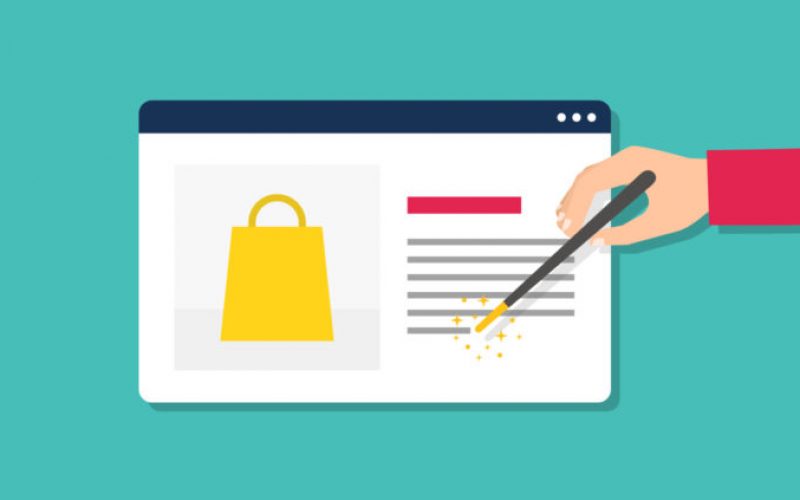
I am trying to write a WordPress plugin and I have hit a bump.
The Background: I have some data in a mySQL DB that I am using (each row has a unique ID and some other information I have added). I am able to search the DB using
$heads = $wpdb->get_results("SELECT * FROM {$wpdb->prefix}costumesdb WHERE location = 'head'", ARRAY_A);
And display some of the information to the user in a form using (this is one of 5 different drop-downs but they are all created in the same way)
<form id="createacostume" action= "admin-ajax.php" method="post">
Head: <select name="head">
<?php foreach ($heads as $head) { ?>
<option value="<?php echo $head['id'] ?>"><?php echo $head['shopName'] . " - " . $head['pieceName'] ?></option>
<?php } ?>
</select>
<input type="submit">
The script to submit the form data to the server is:
<script type='text/javascript'>
jQuery.ajax({
type: "POST",
url: ajax_url,
data: { action: 'my_action' , param: 'st1' }
}).done(function( msg ) {
alert( "Data Saved: " + msg.response );
});
</script>
In my php file serverside is:
add_action('wp_ajax_my_action', 'my_ajax_action_function');
function my_ajax_action_function(){
$reponse = array();
if(!empty($_POST['param'])){
$response['response'] = "I've get the param a its value is ".$_POST['param'].' and the plugin url is '.plugins_url();
} else {
$response['response'] = "You didn't send the param";
}
header( "Content-Type: application/json" );
echo json_encode($response);
exit();
The Problem: If I set action= “admin-ajax.php” in the form I get booted to the admin-ajax.php page with a “0” response.
How do I get data from a form in WordPress admin page and process it?
I worked it out and re-wrote the code:
You have to push onto the form the ajax function you are calling.