Conditionally set post_content in wp_insert_post
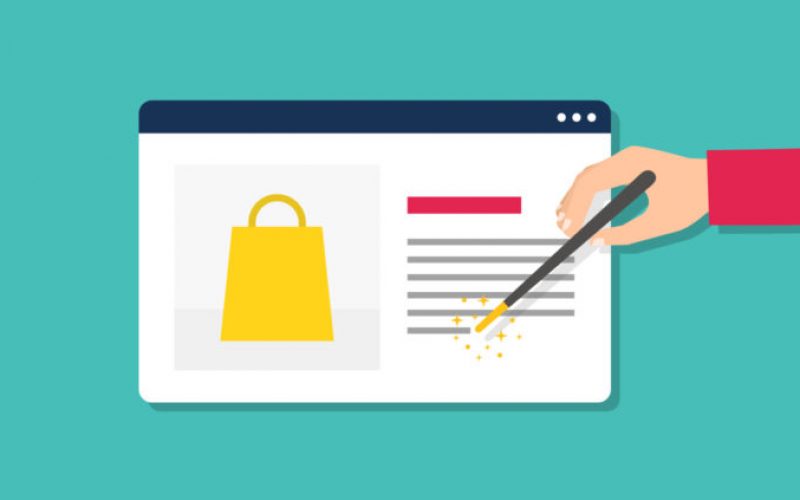
Is it possible to conditionally set the post_content in wp_insert_post?
Im using a foreach to add multiple pages at once:
foreach ($create_pages as $new_page) {
$add_pages = array(
'post_title' => $new_page,
'post_content' => '', // lets say, if the page_title is 'Home', set the post_content to 'Default home page content'
'post_status' => 'publish',
'post_type' => 'page'
);
$page_id = wp_insert_post($add_pages);
}
Would it be also possible to set the default content for all the pages inside a php file?
I can’t help but think I am missing something but what seems like the obvious answer is to alter your
$create_pages
array:It is possible to use a second array of post content and match it against the titles but based on your description of the problem, I can’t see why you’d need that complexity.
Another option would be to use nested arrays, something like this:
I am fairly sure that either version should work fine ( see this and this), though the latter is probably going to be more readable if you have long titles.
If that array is oppressively large, you could put it in another file and
include
it.Yes, you could try this –
Edits:
Regarding a an external logic, you could try the following.
Now, to add the content for a page, ex: About Us
Reference:
Depends on what you want it to be conditional on. E.g.