If a plugin stores data in the usermeta tables what is the best practice method to delete these entries for all users in uninstall.php? I could access the database directly but is there another way?
Best practice way to delete user meta data during plugin uninstall?
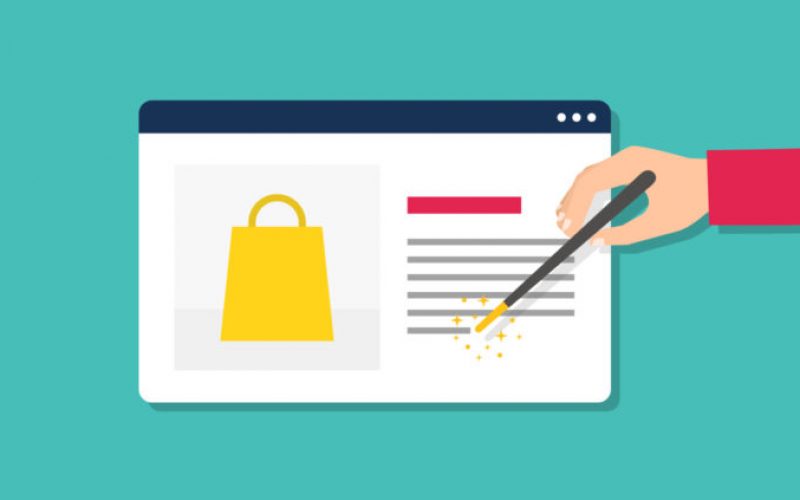
You should not access the database directly, if at all possible. There are two main reasons for this:
If the database structure changes (unlikely), your queries may become outdated. Using functions like
delete_user_meta()
will ensure that your query should work properly for all WordPress versions (past, present, and future) that support that function.Caching. If you don’t clean up the cache after deleting your data, it could cause problems. If you delete your data manually, you also need to clean up the cache manually. Using built in functions that do this automatically is definitely a better idea.
For deleting meta data (such as user meta fields), I would recommend using the
delete_metadata()
function. This function has a fifth parameter that you can set totrue
to remove the metadata with a givenmeta_key
for all objects (in this case users). Example:You can repeat that for each meta key your plugin uses.
It’s best not to interact with the database directly, especially when issuing DELETE statements, since a single typo can destroy unintended data. Instead, use WordPress functions to get a list of all user IDs, then remove the user meta field for each user individually, like so:
Function reference:
http://codex.wordpress.org/Function_Reference/get_users
http://codex.wordpress.org/Function_Reference/delete_user_meta
Best practice is to prefix the meta data your plugin enters that way you can simply do something like a search for all meta_key’s like this
Sample code: