Is there a way to limit the number of words per post through the the_content() function or something similar?
Add Word Limit to Posts
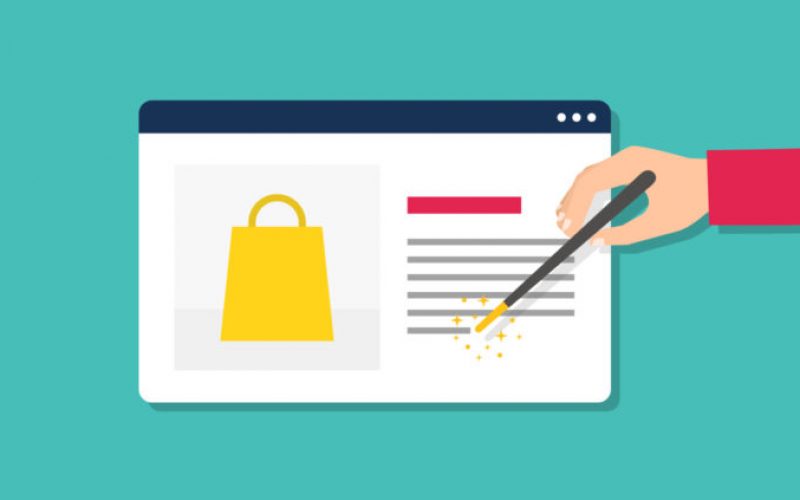
Is there a way to limit the number of words per post through the the_content() function or something similar?
You must be logged in to post a comment.
You can limit is through a filter on
the_content
For example (in functions.php):
Here are a few filters I rely on for limiting word and character counts. The first is character based but only splits between words.
This one excepts a word count variable and looks for your more tag. It also strips out any html before counting then adds it back.