I am using wordpress 3.8. I am using comment_form();
I need to display a success message
on successfully posted comment on blog. How to do this?
Success message in comment form
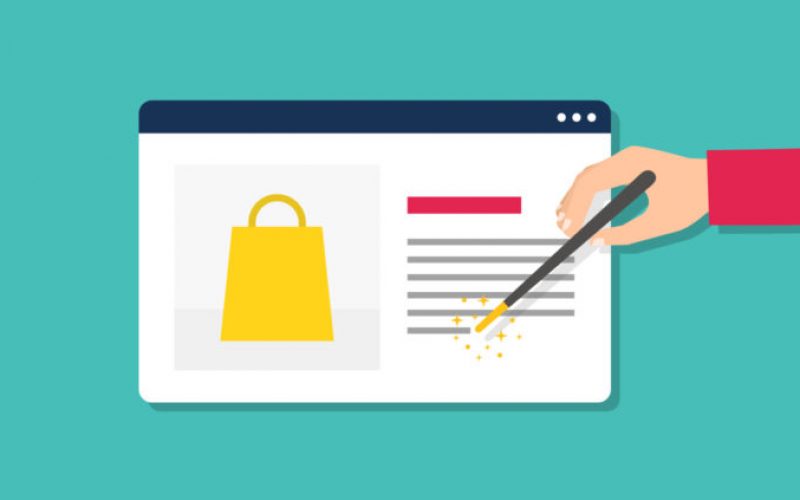
I am using wordpress 3.8. I am using comment_form();
I need to display a success message
on successfully posted comment on blog. How to do this?
You must be logged in to post a comment.
Without Ajax or plugins. Add to function.php:
Try this: http://wordpress.org/plugins/wp-ajaxify-comments/
or (manually)
Add the following lines of code to your theme’s functions.php file
You will need to add two JavaScript files jquery.validate.min.js and ajax-comments.js in the js directory of your theme.
http://bassistance.de/jquery-plugins/jquery-plugin-validation/
and the ajax-comments.js is:
Customize the style of messages
Open your theme’s comments.php and add some CSS classes to comment form input fields as described: To comment author name input, add class=”required” To comment author email input, add class=”required email” To comment author URL input, add class=”url” To the comment textarea, add class=”required”
if you do not want to use AJAX or any plugins, you can use $_GET to understand if comment form has been submitted or not.
In the above case, we have $_GET[‘unapproved’] if you have to approve the comments.
You can consider a statement for avoiding any errors: