I have a custom post and I would like to upload many featured images. I know there are some plugins doing that but I would like to try it without installing a plugin. Is it possible?
Any help/opinion is appreciated
Upload multiple featured images in a custom post (WordPress)
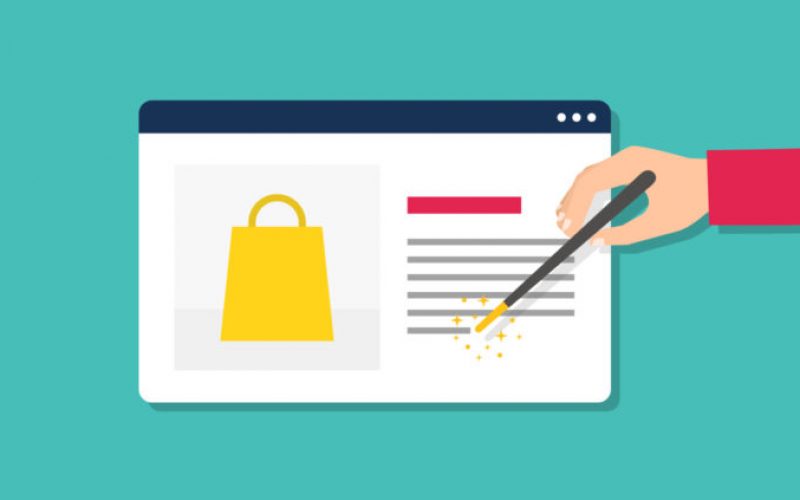
Well, there is only ONE featured image. But you could create a custom meta box where you could add new images. Here’s an example using the wordpress uploader:
functions.php or my-plugin.php:
To create more images you can adjust the
$meta_keys
-Array incustom_postimage_meta_box_func($post)
andcustom_postimage_meta_box_save($post)
To get the saved image ID, you can use
get_post_meta( $post_id, 'second_featured_image', true);
. So maybe like this:<?php echo wp_get_attachment_image(get_post_meta( $post_id, 'second_featured_image', true),'thumbnail'); ?>
Nico Martin wrote a great answer!
I found a few things that, in my opinion, could be improved for a better user experience.
The button and preview styles needed to be adjusted in order to stay consistent with the existing WordPress admin styles, so I added the proper wp classes accordingly. This way any updates to the WordPress admin, and admin themes will work properly.
I added aria text to improve accessibility compatibility.
The original code returned the thumbnail size, which was showing blurry images and cropped images in the preview. I’ve updated the code to return the ‘large’ size, and made it visually simpler to use any size you prefer by changing the second parameter of
wp_get_attachment_image_src( $image_meta_val , 'large')
.I added an hr element to provide better visual separation between the images.
I adjusted to text to reflect the current featured-image button text.
I changed the js and PHP to better hide/show the containers and buttons.
I added more images, as I needed them for my project. These can easily be adjusted by adding more names to or removing names from the $meta_array. Remember that any changes made to this value need to be made in both places the variable occurs.
This should provide a more consistent flow visually and a better user experience all around.
functions.php or my-plugin.php:
To get the saved image URL, height, and width, and display the image in your theme, you can use something like this:
Replace the second parameter of
get_post_meta( $post_id, 'featured_image_2', true)
with any of the names that you used in the $meta_array to get that image.