How to add audio files to wordpress blog that when someone clicks on a post Music began playing by itself.
How to add audio files to wordpress blog and making it auto play?
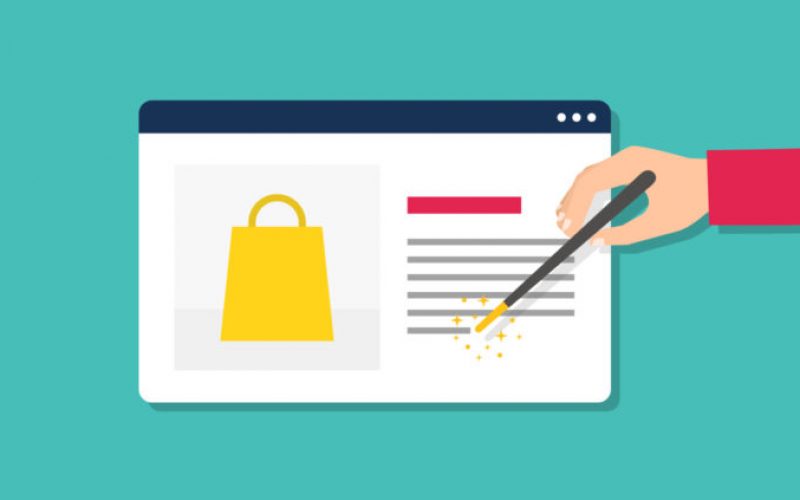
How to add audio files to wordpress blog that when someone clicks on a post Music began playing by itself.
You must be logged in to post a comment.
Basic parts
To get all audio files attached to a post use
get_children()
:For the last attached file URL use
wp_get_attachment_url()
and pass the ID of the last child as an argument:To insert an audio player use the HTML5
audio
element with anautoplay
attribute:There are other solutions to play audio files with a better compatibility. Iâll leave it up to you to implement those.
The plugin
Letâs put this together. I recommend to use this a plugin so you can turn it off fast once your visitors start complaining. That will happen soon, see the caveats at the end of this answer. 🙂
Uploading audio files
By default WordPress does not allow uploading all files. A
wav
for example will be rejected. You might need another plugin to extend the list of allowed mime (file) types:Caveats
Please add a warning in your post list. Do not omit the controls. Many visitors will not enjoy auto play sounds because they â¦