i’m trying to make an android native app for my WordPress blog. I heard that WordPress has JSON-API to handle the information of a blog but I don’t know how I could use it. Please, could someone give me a tiny introduction of its use and if I could use the GSON library to manage the information within my Android app?
Thanks!!
Android- App with WordPress API e GSON
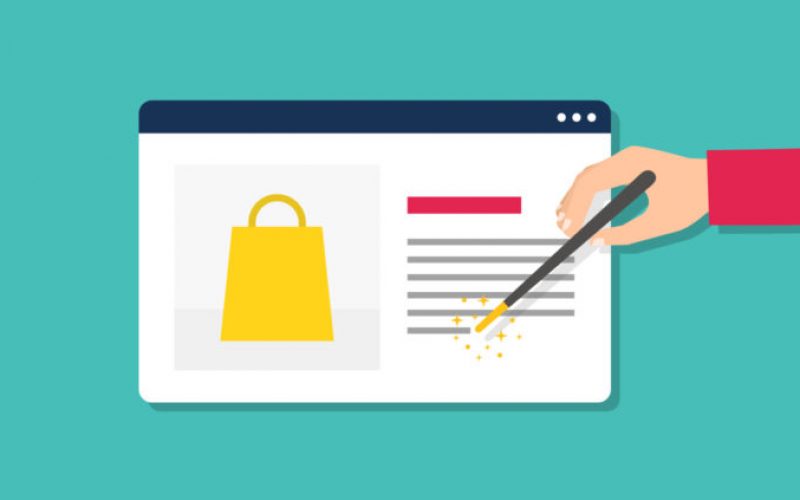
Simple example to parse/deserialize your JSON string
Refer Gson API documentation for more details. Hope this will be helpful.
To use GSON you will have to create a class with the exact same fields as the JSON you will want to parse. GSON till then take in a JSON string and instantiate an object with the data. For example if you have some JSON with som information like:
and you would like to parse that. You have to create a class like:
And then use GSON:
and the p object will have the data from your JSON string.