Upload Base64 Image Facebook Graph API
i want to use this script that link is attached how i can use this in my wordpress post?
i want to use this for fbcover photo site.
upload base64 image facebook graph api how to use this script
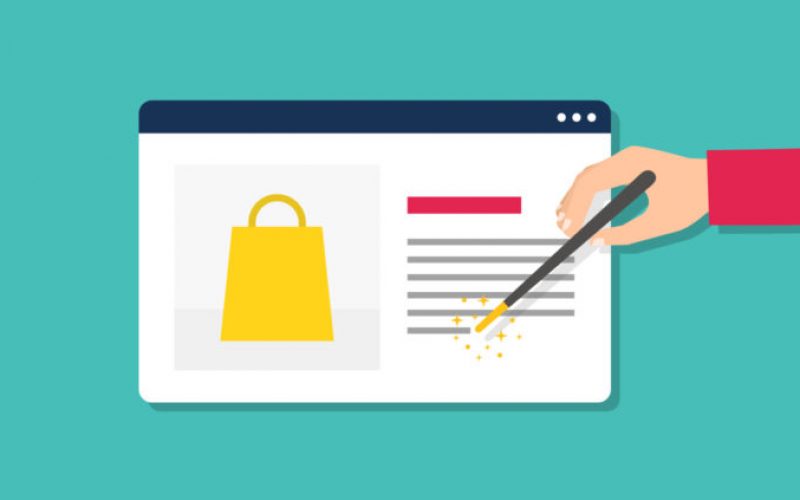
Upload Base64 Image Facebook Graph API
i want to use this script that link is attached how i can use this in my wordpress post?
i want to use this for fbcover photo site.
You must be logged in to post a comment.
Take a look at this code I hacked together from various examples – you can use this to post a pure base64 string to the Facebook API – no server side processing.
Here’s a demo: http://rocky-plains-2911.herokuapp.com/
This javascript handles the converting of a HTML5 Canvas element to base64 and using the Facebook API to post the image string
This handles the Facebook Authentication and shows basic HTML setup
I needed this too, and was not happy with all the code around it because it is lengthy and usually needs jQuery. Here is my code for uploading from Canvas to Facebook:
Source: http://www.devils-heaven.com/facebook-javascript-sdk-photo-upload-from-canvas/