how to get a page id in wordpress using jquery alone.I am planing to change some styles of a page using a custom script for which i need to know page id.
how to get the current page id in wordpress using jquery
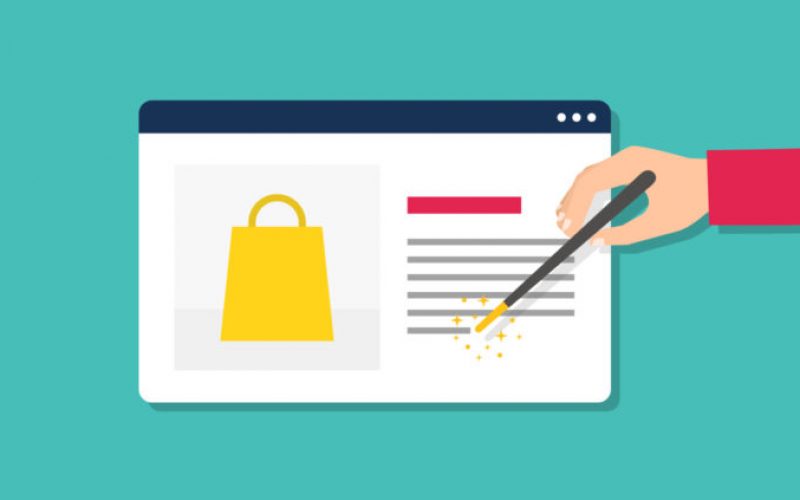
how to get a page id in wordpress using jquery alone.I am planing to change some styles of a page using a custom script for which i need to know page id.
You must be logged in to post a comment.
Add this function to your code.
You can now get the page id by using:
Use
wp_localize_script
.You can use the vaiables in your scripts this way..
If you want to get the current page id in jQuery alone you can do it in following steps:
c_pageid
and the valueget_the_ID();
var pageId=$("#c_pageid").val();
This may solve your problem.
The best way to do this is by adding global javascript variables via PHP.
To do this first add the following script to your page.php template file:
Now in in your javascript code you are able to use this global variable like this.
You can use this same technique to use other WordPress variables in javascript.
Use the above line in your script