I have a arbitrary string and want to check if a post with that slug string exists in the site. I tried to find a way to list all slugs, but can’t find such a thing. Thanks
How to check if a slug exists?
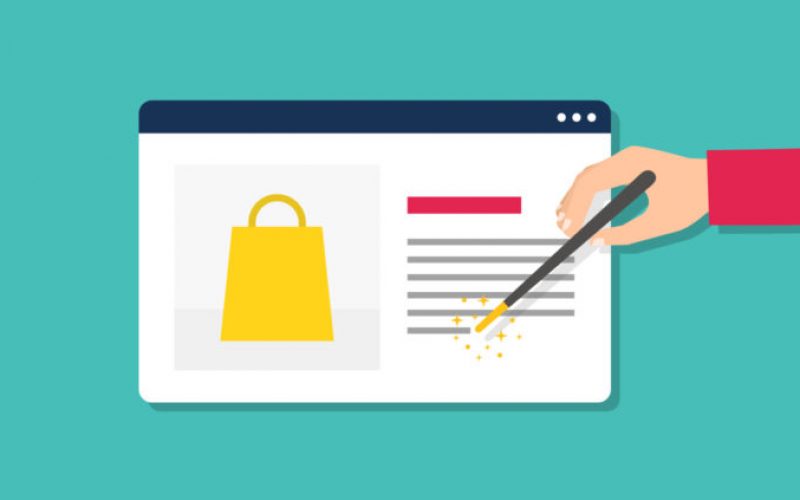
I have a arbitrary string and want to check if a post with that slug string exists in the site. I tried to find a way to list all slugs, but can’t find such a thing. Thanks
You must be logged in to post a comment.
This is what you’re looking for, tested and I use it on my own sites:
You can then use it like this:
Replace
contact
with whatever slug you want to test for.Do you mean post slug? You can try to make use of
wp_unique_post_slug()
that WP uses to generate those. If I remember right if slug you are trying to use is not unique it will be returned with numerical index appended.How about this simpler approach?
If a post doesn’t exist for the given slug and post type provided then
get_page_by_path()
returns null.You then have more than enough information to test if a post was returned or not, hope this helps.